In the previous tutorial we had a homing missile chasing after a single target. This tutorial will show you how to convert your homing missiles into heat-seeking missiles for multiple targets.
If you haven’t read the first Homing Missile tutorial, you may download this .zip file, which contains the source code we’ll be starting with on this tutorial.
Final Result Preview
Let’s take a look at the final result we will be working towards:
Step 1: Modify the Cannon Graphic
The only Movie Clip in the Library we’ll need to change is the Cannon, since we’ll make it aim at the closest target before shooting. Remember that 0° of rotation means pointing at the right, so make the graphic accordingly.
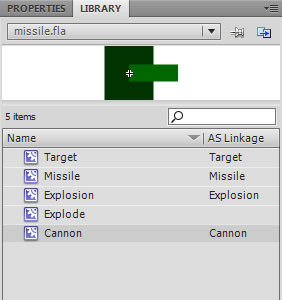
Step 2: Declare Distance Variables for the Cannon
I’m going to reuse the targetX and targetY variables to calculate the distance of the cannon from the target, so I’ll declare them at the beginning of the class instead of inside the playGame function, as well as a new variable to store the calculated distance:
private var missile:Missile = new Missile(); private var speed:int = 15; private var cannon:Cannon = new Cannon(); private var missileOut:Boolean = false; private var ease:int = 10; private var target:Target = new Target(); private var floor:int = 385; private var gravity:Number = 0.5; private var targetVY:Number = 0;//Current vertical velocity of the target private var distance:int; private var targetX:int; private var targetY:int;
Now the targetX and targetY variables will be already declared for the playGame function:
private function playGame(event:Event):void { if (missileOut) { if (missile.hitTestObject(target)) { var explosion:Explosion = new Explosion(); addChild(explosion); explosion.x = missile.x; explosion.y = missile.y; removeChild(missile); missileOut = false; } else { targetX = target.x - missile.x; targetY = target.y - missile.y; var rotation:int = Math.atan2(targetY, targetX) * 180 / Math.PI; if (Math.abs(rotation - missile.rotation) > 180) { if (rotation > 0 && missile.rotation < 0) missile.rotation -= (360 - rotation + missile.rotation) / ease; else if (missile.rotation > 0 && rotation < 0) missile.rotation += (360 - rotation + missile.rotation) / ease; } else if (rotation < missile.rotation) missile.rotation -= Math.abs(missile.rotation - rotation) / ease; else missile.rotation += Math.abs(rotation - missile.rotation) / ease; var vx:Number = speed * (90 - Math.abs(missile.rotation)) / 90; var vy:Number; if (missile.rotation < 0) vy = -speed + Math.abs(vx); else vy = speed - Math.abs(vx); missile.x += vx; missile.y += vy; } } targetVY += gravity; target.y += targetVY; if (target.y > floor) { target.y = floor; targetVY = -18; } }
Step 3: Make the Cannon Point Towards the Target
[...]
Getting Warmer: Smart Aiming With Heat-Seeking Missiles
No comments:
Post a Comment