The Concreteness of Abstraction
The first time I went to London, I picked up a Tube Map (Map of the London Underground) and was able to get where I wanted to go. The map of London’s tube is a masterpiece of clarity and abstraction. Based on electrical circuitry, it’s very easy to find one’s way around [...]
Best articles on Flash Development and Software Design
Tuesday, May 31, 2011
Beginning ActionScript 3.0 Abstraction : The Factory Method at Work
Monday, May 30, 2011
QuickB2
QuickB2 is a complete abstraction of Box2D, providing grouped hierarchies, extensible classes, and event-driven callbacks. The high-level interface enables developers to create physics simulations that are impractical to write using Box2D’s API alone.
Some features:
- Soft-bodies.
- Top-down car physics.
- Friction in z-direction (top-down friction).
- Event-driven through revent.
- Retain mode that doesn’t require destroying/remaking objects.
- Extensible architecture.
- Nested hierarchy system similar to Flash’s display hierarchies.
- Cloneable objects.
- Highly customizable immediate-mode rendering for both debug and production purposes.
- Hooks for integration with any typical retain-mode-type rendering engine (e.g. the Flash display list).
- Various debugging tools including visualizations, mouse dragging, warnings, notifications, asserts, exceptions, and runtime GUIs.
- Pixel-based units.
- AABB and circular bounding representations.
- Easy low-level access to Box2D API in case of emergency.
- Large suite of “stock” functions and classes to address common needs.
- Individual polygons may be concave and have any number of vertices.
- All kinds of joints, including built-in spring joints.
- Physics world is completely changeable within contact callbacks.
- WYSIWYG editing environment for Flash CS5.
- Built-in system for handling your game loop and time steps.
- Easy API for beginners – lower-level optimizations and tricks available for advanced users.
Thursday, May 26, 2011
Generating Digital Audio Using SiON
In this tutorial I’ll be showing you how to get started with SiON, an AS3 software synthesizer library which generates sound using only code.
Final Result Preview
In the end this is what we’re going to obtain:
Click on the darker rectangle area to start/stop the balls movement.
Getting Necessary Files
First you need to get the SiON library. You can download it either as a SWC file or as uncompressed ActionScript files. To do this go to SiON Downloads and select the desired download method.
After you’ve downloaded the source code add it to your global class path.
Notice that on this page you can also download the ASDoc documentation and older versions of the library.
In this tutorial we’ll make use of the well known minimalcomps library, developed by Keith Peters; if you don’t have it go ahead and grab it: minimalcomps.
Also add the minimalcomps library to your global class path and let’s get started.
Note: As always I’ll be using FlashDevelop throughout this tutorial. You can use whatever code editor you like although I recommend sticking with FlashDevelop.
Step 1: What is SiON?
The SiON library is a software synthesizer library built in ActionScript 3.0 and works in Flash Player 10 or higher.
With SiON you can generate dynamic sounds on the run without the need of loading any audio files. Also makes it very easy to synchronize sounds with display objects (eg. object hitting a wall, explosion etc).
From the multitude of features it has I’ll show you the essentials of working with it: using MML (Music Macro Language) data to generate sound, using voice presets and effectors on playing sounds, setting the tempo (BPM), panning and changing volume and lastly I’ll show you how to sync sounds with display objects.
Step 2: Setting up the Project
Let’s start by creating a new project. Open your code editor and create a new ActionScript 3 project [...]
Read more: Generating Digital Audio Using SiONWednesday, May 25, 2011
Detecting Key Combos, the Easy Way
Have you ever been amazed at the variety of attacks in fighting games like Mortal Kombat, Super Smash Bros, Soul Calibur and others? Now you can learn how to create an engine to detect key combinations and build your own fighting game as well!
Final Result Preview
Let’s take a look at the final result we will be working towards:
The combos in this demo are: ASDF
, AAA
, and SSS
. Type them!
Step 1: Introduction
Ever wanted to build a fighting game (or any other genre) with lots of combos? In this tutorial we will create a simple class to detect key combos and tell us when a combo has been done by the user. We will also create a very simple graphical interface to let us test our class.
Step 2: Starting a New Project
For this tutorial, we will use FlashDevelop‘s pure AS3 project with preloader. We will create a project with a preloader only to make it easy for you if you want to keep working on the final result towards a game. Let’s start by opening FlashDevelop and selecting our project:
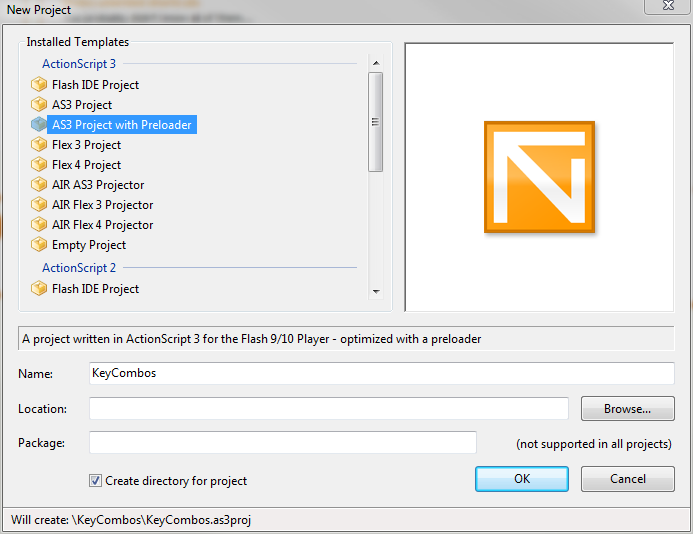
With that, we can begin working on our classes.
In order to use the graphics we’ll create in Flash Pro within our AS3 project, we need to export our images from the .fla file to a .swc format. More information about this format can be found in Option 2 of this guide to FlashDevelop. Create a new AS3 FLA in Flash Professional, then change the settings on our .fla file to export its content to a .swc format: go to File > Publish Settings (or press Ctrl+Shift+F12) and check the “Export SWC” box under the “Flash” tab.
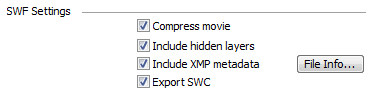
If you don’t have Flash Professional, don’t worry. I’ve included the final SWC file in the download package for this tutorial. Download it, then skip to Step 6.
Step 3: The Basic Shape of the Button
We will first create all the graphical part and worry only with the code later. Since we will be dealing with key combos, let’s create a button with a letter in it to represent a key. Our button will be very simple: three circles with different colors and some filters in it. That’s how I built mine: a big gray circle with a white circle on top of it, and a red circle on top of the white one. After that, I applied a glow and two drop shadow filters on the red circle in order to get the final result, which is included in the source files.

For more details on how the button was built, grab the source files for this tutorial!
Step 4: Up and Down Images
We now have to give our button “up” and “down” images. Before doing that, we need to turn it into a symbol. Let’s convert it to a symbol, give it a name of KeyButtonImage
and export it as “SWCAssets.KeyButtonImage”. We are adding the SWCAssets
package in the class name for organization purposes when we start coding. This will be more clear later [...]
Creation of a platform game using FlashPunk
During these days I am playing a bit with FlashPunk and I want to share with you a couple of examples.
We are going to build a simple platform game.
Getting the required software
While most developers use Flash Builder or FlashDevelop, I will use Flash Professional CS5.5, so the first thing you should do is getting a copy of it. You can get a fully functional 30 days trial at this page.
Then, download the latest release of FlashPunk from this page, and you are ready to go.
Preparing the worspace
All in all, FlashPunk is just an AS3 library, and although it has been developed for being used outside the Flash IDE, you just have to copy the net
foder into your project folder and you are ready to go.
Hello FlashPunk
At this time in your main class (I called mine punk
), enter this code:
1 2 3 4 5 6 7 8 9 | package { import net.flashpunk.Engine; import net.flashpunk.FP; public class punk extends Engine { public function punk() { super(640,480,60,false); } } } |
I said FlashPunk has been developed for being used outside the Flash IDE, and that’s it… we haven’t Sprites or Movieclips, but the class extends Engine
FlashPunk’s class.
This line
6 | super(640,480,60,false); |
Calls the constructor of the extended class, that is Engine
constructor, with these arguments:
width: the width of your game in pixels
height: the height of your game in pixels
frameRate: the game framerate, in frames per second (default: 60)
fixed: true if a fixed-framerate should be used, false (default) otherwise.
Test the movie and you will see a dark background color, no matter the Stage color.
Your FlashPunk project works!
Adding some walls
When it’s time to add graphics to our FlashPunk games, we have to draw them with our preferred paint software. Using Photoshop, I made a 32×32 pixels square and called saved it as wall.png
in a newly created assets
folder in the same level of net
(the one which contains FlashPunk library).
Then change punk.as
this way:
Thursday, May 19, 2011
Flash procedural perfect cave generation
Today I’ll show you a procedural way to generate perfect caves, to be used in roguelike games.
The roguelike is a sub-genre of role-playing video games, characterized by randomization for replayability, permanent death, and turn-based movement. Most roguelikes feature ASCII graphics, with newer ones increasingly offering tile-based graphics. Games are typically dungeon crawls, with many monsters, items, and environmental features. Computer roguelikes usually employ the majority of the keyboard to facilitate interaction with items and the environment. The name of the genre comes from the 1980 game Rogue (source).
Please note I said “perfect cave” but I do not mean “better than the rest”, but a cave in which every tile can be reached from any other tile without jumping through walls.
This is the code [...]
Read more: Flash procedural perfect cave generationWednesday, May 18, 2011
Build a Minesweeper Game Within 200 Lines of Code
In this tutorial we will learn how to plan and code a minesweeper game using AS3 and Flash. Can we make it in just 200 lines?! Let’s see…
Final Result Preview
Let’s take a look at the final result we will be working towards:
Because Flash Player doesn’t support right-click, shift-click on the squares you think contain mines in order to mark them.
Minesweeper Rules
If you’re a minesweeper player, then probably you know the game rules — but just in case you’re not:
Minesweeper game consists of a group of squares where each square represents a region. Each region either contains a mine or not. Your mission is to reveal (or “open”) all the mine-free regions and mark the ones that contain mines. You open the region by left-clicking it, and mark a region by shift-clicking. The key for determining whether a certain region contains a mine or not is that for every 3×3 grid (9 regions) of squares, the number written on the region in the center represents the number of mines in the 8 other surrounding regions.
So, when starting your game, your first few clicks will depend on your luck to find mine-free regions, but once you open a fair amount of regions, you can use logic to find the regions that contain mines.
A good example for a minesweeper game, is the one that ships with Windows (any version); a simple search for [online minesweeper game] will also direct you to several versions, which all have the same concept. Otherwise, play the game from the final SWF file, embedded above.
Modeling Minesweeper the OOP Way
In a minesweeper game, if we want to think of the game from the OOP point of view, we can consider each region as an object. We will call this object a ‘zone’, and all of the game zones will be in a container object that we will call the ‘board’.
For every zone object, there is a bunch of properties like:
- state: whether it contains a mine or not.
- revealed: if it’s revealed, the user has opened the zone.
- marked: whether the user has marked the zone as containing a mine.
- zoneValue: the number written inside the zone representing the number of mines in the surrounding zones.
- xcor: the horizontal position of the zone in the board.
- ycor: the vertical position of the zone in the board.
And that’s how we will build the game. there will be a Zone
class linked with a zone movie clip in the library, and we will instantiate the amount of zones we need from our main class to make the game board.
Addressing the Right-Click Problem within Flash
For a regular minesweeper game — a native one that’s not in the browser — you can have access to both mouse buttons, so that the user playing the game can open regions by left clicking and mark them by right clicking. If you were to publish the game as an AIR application, then you would have access to all of the mouse features including the right and middle mouse buttons, and you should do that for better user experience while playing the game.
But if you want to publish the game via Flash Player in the browser, then you don’t have access to the right mouse button, since the right mouse button will open the context menu for the Flash Player; so we have to figure out a solution for this problem.
The solution that I came up with is through using the keyboard. I made it by detecting the shift key, and found that the easiest way to do that is by using the shiftKey
property for the MouseEvent
class. there are a bunch of other properties for some special keyboard keys like the Alt (for windows), Option (for mac), and the Ctrl (Command) key. I recommend that you check them out in the documentation help for the MouseEvent class if you don’t already know them.
Contents of the FLA
Let’s take a look at the FLA that we will be working with.
[...]
Read more: Build a Minesweeper Game Within 200 Lines of CodeTuesday, May 17, 2011
Beginner’s guide to SWF internals
I have recently pushed the initial version of a new framework – the AS3Introspection library – up to github. AS3Introspection can decode a SWF and generate a representation – by default as XML – of its code contents. Whilst writing an introduction to AS3Introspection, it occurred to me that for someone to understand how it [...]
Read more: Beginner’s guide to SWF internalsPatternCraft – Adapter Pattern
PatternCraft is a series of video tutorials which uses Starcraft references to teach Design Patterns. This tutorial covers the Adapter Pattern by showing how to add Mario and Sonic to Starcraft [...]
Read more: PatternCraft – Adapter PatternUsing cellular automata to generate random land and water maps with Flash
After the first attempt to generate land and water maps using random numbers, it’s time to try to do the same thing using cellular automata.
A cellular automaton (plural: cellular automata) is a discrete model studied in computability theory, mathematics, physics, complexity science, theoretical biology and microstructure modeling.
It consists of a regular grid of cells, each in one of a finite number of states, such as “On” and “Off” (or “Land” and “Water”).
The grid can be in any finite number of dimensions. For each cell, a set of cells called its neighborhood (usually including the cell itself) is defined relative to the specified cell.
For example, the neighborhood of a cell might be defined as the set of cells a distance of 2 or less from the cell.
An initial state (time t=0) is selected by assigning a state for each cell. A new generation is created (advancing t by 1), according to some fixed rule (generally, a mathematical function) that determines the new state of each cell in terms of the current state of the cell and the states of the cells in its neighborhood.
For example, the rule might be that the cell is “On” in the next generation if exactly two of the cells in the neighborhood are “On” in the current generation, otherwise the cell is “Off” in the next generation.
Typically, the rule for updating the state of cells is the same for each cell and does not change over time, and is applied to the whole grid simultaneously, though exceptions are known (source).
What does this mean? In simple words, we are placing random land and water tiles in a map, and let the cellular automaton (read carefully: “automaton” not “automation”) draw the map according to a set of predefined rules.
So this is an example of a starting map, generated with random tiles of water and land, with each tile having the same probability of being land or water [...]
Read more: Using cellular automata to generate random land and water maps with FlashMonday, May 16, 2011
Understanding the Game Loop – Basix
Almost every game can be thought of as having one main function that contains all the game logic, and which is run either when the user does something, or after a certain amount of time. This cycle of running the same core function over and over again is called the game loop, and is crucial to understand for any sort of game development.
You’ve probably played the board game Chutes and Ladders (or Snakes and Ladders as we call it in the UK).
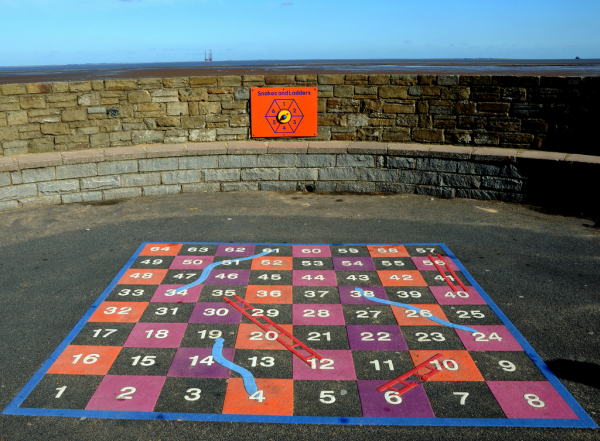
(Photo credit incurable_hippie on Flickr)
Each player rolls the die (or spins the spinner) and moves forward the number of squares indicated. The square they land on may direct them to slide backwards or climb forwards several spaces. The player wins the game when they get to the final square.
So, in the above image, landing on Square 6 makes you climb four squares to Square 10; landing on Square 19 makes you slide back 15 squares to Square 4; and landing on Square 64 means you win the game.
Now suppose you’re playing single player, to practice. You do the same thing as above, over and over again, until you reach Square 64. How would you represent this in code?
You’d probably start by creating an array to store the values of the squares. Most elements would contain zero, but a few would contain either a positive number (indicating a ladder), or a negative number:
Note: This is pseudocode, not AS3
var specialSquares:Array = []; specialSquares[0] = 0; //the square the players start on, before 1 specialSquares[1] = 0; ... specialSquares[6] = +4; ... specialSquares[19] = -15;
…and so on. Then, you’d have a function to move the player based on the number on the die:
function movePlayer(squares:Number) { newSquare = currentSquare + squares; newSquare += specialSquares[newSquare]; currentSquare = newSquare; }
You could then put this inside a bigger function representing a whole turn:
function takeATurn() { diceNumber = random(1, 6); //random number between 1 and 6 movePlayer(diceNumber); if (currentSquare == 64) { //player has won! game over } }
This function, takeATurn()
, encapsulates the core game logic for single-player Chutes and Ladders. But how do we actually get this function to run? There are several options:
Run the Function Based on Player Input
[...]
Read more: Understanding the Game Loop – BasixPatternCraft – The Differences Between the Bridge, State, and Strategy Patterns
I’ve already covered the state pattern and the strategy pattern, but many people find it difficult to distinguish between the two. I put this tutorial together to help highlight the differences. I also included the bridge pattern because, although it’s not a “behavioral pattern”, it’s usage is very similar and helps show how different these [...]
Read more: PatternCraft – The Differences Between the Bridge, State, and Strategy Patterns3D car with a camera that follows it
Ever wondered how to build a simple 3D car movement with camera that follows the car?
Let’s have at this tutorial, which uses Alternativa 3D 8 (Molehill).
Download Alternativa 3D 8.
This tutorial consist of these parts:
- move a car with keyboard controls
- follow the car with a camera
The final app will look like this:
(*requires Flash Player with Molehill)
To understand the car’s movement let’s do a little bit of math.
Speed / Acceleration
When we press UP key, we add acceleration to the speed. When DOWN key, we subtract acceleration from the speed. When none of them is pressed, we slow down by multiplying actual speed by 0.96 – note it can be whatever, depends on how fast or slow you want to slow down.
// don't allow to speed up more than maximum speed if (accelerate && speed<speedMax) { speed+=2; }else if (brake) { speed-=2; }else{ // When the speed is below 0.3 threshold, // we pass 0 to stop the car // and avoid little unwanted movements. if (Math.abs(speed)>0.3) { speed*=0.96; } else { speed=0; } }
Steering / Rotation
When we press LEFT or RIGHT key, we turn the car by Math.PI/40 radians (4.5 degrees). Again, pass number that fits you best.
[...]
Read more: 3D car with a camera that follows itThursday, May 5, 2011
Flash physics draw game with a car using QuickB2
QuickB2 is a complete abstraction of Box2D, providing grouped hierarchies, extensible classes, and event-driven callbacks.
The high-level interface enables developers to create physics simulations that are impractical to write using Box2D’s API alone.
It takes care of things that you don’t want to, so that you can concentrate on your game.
Among its top features we can find:
* Soft-bodies.
* Top-down car physics.
* Friction in z-direction (top-down friction).
* Pixel-based units.
* Individual polygons may be concave and have any number of vertices.
* All kinds of joints, including built-in spring joints.
* WYSIWYG editing environment for Flash CS5.
Combining one of the official examples with the drawing concept introduced during the creation of Way of an Idea Box2D prototype I ended up with this script [...]
Read more: Flash physics draw game with a car using QuickB2Tuesday, May 3, 2011
Game Design Logs
If you still practice or encourage the outdated practice of writing long design documents, you are doing your team and your business a grave disfavor. Long design docs embody and promote an insidious world view: They make the false claim that the most effective way to make a game is to create a fixed engineering specification and then hand that off to developers to implement feature by bullet-pointed feature.
Great game development is actively harmed by this assumption. Pre-allocating resources at an early stage interrupts the exploratory iteration needed to find the fun in a game. A written plan that stretches months into the future is like a stake through the heart of a good game process. Instead of quickly pivoting to amplify a delightful opportunity found during play testing, you end up blindly barreling towards completion on a some ineffectual paper fantasy.
Yet, there is still a need for documentation. Why?
- We need a persistent repository of decisions: Teams include many people and conversation occurs asynchronously. Without centralized documents, you end up with a fragmented conversation where many decisions made in one-on-one conversations are lost to the broader team forever.
- We need a shared vision: Documents also helps forge a common vision of the next iteration. In a situation where everyone has strong and varied opinions, it is essential that someone can lead the team to by unambiguously stating what comes next. Apparently even God needed documentation.
Design logs
What I do now is write a little something I call a 'design log.' Game design is a process of informed iteration, not a fixed engineering plan that you implement. The form of your design documentation should flow from this philosophy [...] Read more: Game Design LogsMonday, May 2, 2011
Flash Game Dev Tip #9 – Dealing with a lack of motivation to finish your game
Tip #9 – Dealing with a lack of motivation to finish your game
You are not alone
Everyone suffers from this problem at one point or another: You simply can’t face the thought of working on your game. This is especially hard when making the game in your “free time”. It’s all too easy to think “what the hell” and double-click that Team Fortress icon instead of FlashDevelop. And even days when your head is saying “let’s code!” all that motivation can vanish the moment you open the source and realise that’s been so long since you were last here, it’s like staring at a foreign language, or a giant brick wall and you have no idea what to start first.
There is no “single solution” to fix this. It’s extremely personal. Your reasons for not wanting, or being able to code will be as varied as the number of people reading this. But as I said at the start you are not alone – so I posed the question of how to deal with it to 13 fellow game developers and collated their replies. Hopefully somewhere in these insights you’ll find a technique or suggestion that helps you out. And if you have one that you personally use, not already mentioned, please post it into the comments.
I’ll kick things off …
Read more: Flash Game Dev Tip #9 – Dealing with a lack of motivation to finish your gameIndexing Fields Is Really Slow
One of the very nice features of AS3 (and AS2 and JavaScript for that matter) is that you can dynamically access the fields of any object. This leads to much more dynamic code since you no longer need to know what field to access at compile time. As we’ve often seen with other dynamic features, [...]
Indexing Fields Is Really Slow