Almost every game can be thought of as having one main function that contains all the game logic, and which is run either when the user does something, or after a certain amount of time. This cycle of running the same core function over and over again is called the game loop, and is crucial to understand for any sort of game development.
You’ve probably played the board game Chutes and Ladders (or Snakes and Ladders as we call it in the UK).
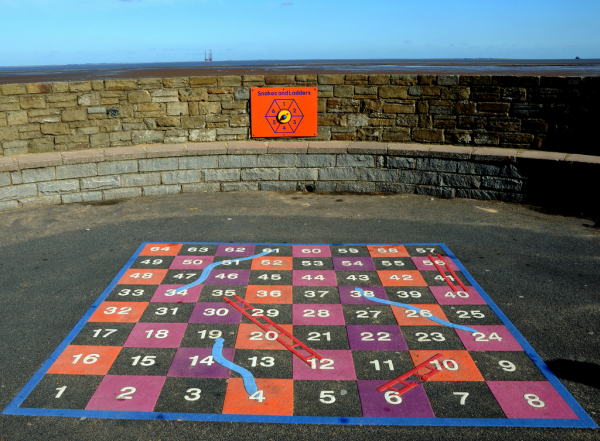
(Photo credit incurable_hippie on Flickr)
Each player rolls the die (or spins the spinner) and moves forward the number of squares indicated. The square they land on may direct them to slide backwards or climb forwards several spaces. The player wins the game when they get to the final square.
So, in the above image, landing on Square 6 makes you climb four squares to Square 10; landing on Square 19 makes you slide back 15 squares to Square 4; and landing on Square 64 means you win the game.
Now suppose you’re playing single player, to practice. You do the same thing as above, over and over again, until you reach Square 64. How would you represent this in code?
You’d probably start by creating an array to store the values of the squares. Most elements would contain zero, but a few would contain either a positive number (indicating a ladder), or a negative number:
Note: This is pseudocode, not AS3
var specialSquares:Array = []; specialSquares[0] = 0; //the square the players start on, before 1 specialSquares[1] = 0; ... specialSquares[6] = +4; ... specialSquares[19] = -15;
…and so on. Then, you’d have a function to move the player based on the number on the die:
function movePlayer(squares:Number) { newSquare = currentSquare + squares; newSquare += specialSquares[newSquare]; currentSquare = newSquare; }
You could then put this inside a bigger function representing a whole turn:
function takeATurn() { diceNumber = random(1, 6); //random number between 1 and 6 movePlayer(diceNumber); if (currentSquare == 64) { //player has won! game over } }
This function, takeATurn()
, encapsulates the core game logic for single-player Chutes and Ladders. But how do we actually get this function to run? There are several options:
Run the Function Based on Player Input
[...]
Read more: Understanding the Game Loop – Basix
No comments:
Post a Comment