Sometimes you have a set of items — could be Strings, could be Numbers, could be Objects, whatever — whose order you want to randomise. This is particularly useful for quizzes and games of chance, but comes in handy in all sorts of other applications. The easiest method I’ve found for doing this is to stick all the items in an Array and then shuffle it like a deck of cards. But how can we do that…?
As a simple example, we’ll use the letters of the alphabet:
var letters:Array = ["A", "B", "C", "D", "E", "F", "G", "H", "I", "J", "K", "L", "M", "N", "O", "P", "Q", "R", "S", "T", "U", "V", "W", "X", "Y", "Z"];
There are different approaches we can take to actually sorting this array.
The Naive Approach
We could create a second array, and copy each element of the first into a random position in the second:

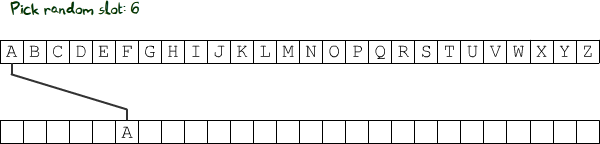
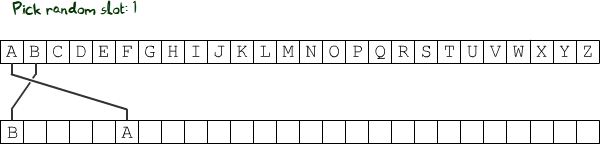
The code for that might look like this (see this Quick Tip on getting a random integer within a specific range for more details):
var letters:Array = ["A", "B", "C", "D", "E", "F", "G", "H", "I", "J", "K", "L", "M", "N", "O", "P", "Q", "R", "S", "T", "U", "V", "W", "X", "Y", "Z"]; var shuffledLetters:Array = new Array(letters.length); var randomPos:int = 0; for (var i:int = 0; i < letters.length; i++) { randomPos = int(Math.random() * letters.length); shuffledLetters[randomPos] = letters[i]; }
But there’s one huge problem. What if the random position picked for C
is 6 as well?
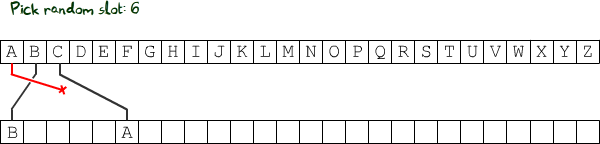
Here, A
gets overwritten, and will therefore not be in the shuffled array. That’s not what we want, so we need to check that the slot is empty before copying the letter over, and pick a different slot if it isn’t: [...]
No comments:
Post a Comment